Ever get hooked on an idle clicker game like Cookie Clicker? These games are simple but addictive, and they’re a great way to learn basic web development. In this step-by-step guide, we’ll walk you through creating your own beer brewing clicker game using HTML, CSS, and JavaScript.
By the end, you’ll have a working game where players can brew beers, buy upgrades, and grow their beer empire. Whether you’re new to coding or just looking for a fun project, this tutorial has you covered.
The code for this project will be available both here and on GitHub.
The Beer Brewing Clicker game is a simple and fun project that shows how to build an idle clicker game from scratch using HTML, CSS, and JavaScript. The goal is to brew as many virtual beers as possible by clicking a button and then use those beers to buy upgrades that increase your brewing rate. You’ll start with just a single click, but as you progress, you can unlock upgrades that automate the process, boosting your “Beers Per Second” (BPS). The game includes features like auto-saving your progress, responsive design, and a clean layout that makes it easy to expand.
I’ll be using some frameworks, mainly Tailwind for the CSS and Alpine.js for parts of the JavaScript.
Let’s get down to it, and we’ll begin this first part with a dead simple layout and functionality. This is what we’re making:
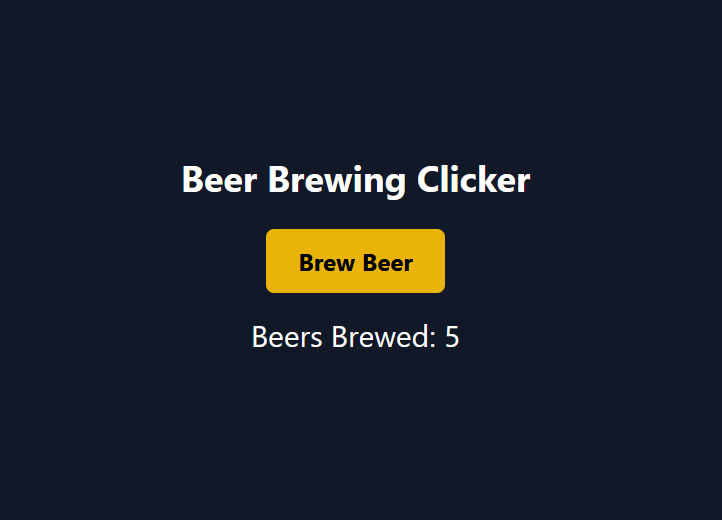
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Beer Brewing Clicker</title>
<!-- Tailwind CSS -->
<script src="https://cdn.tailwindcss.com"></script>
<!-- Alpine.js -->
<script src="https://cdn.jsdelivr.net/npm/alpinejs@3.12.0/dist/cdn.min.js" defer></script>
</head>
<body class="bg-gray-900 text-white flex items-center justify-center min-h-screen">
<div x-data="{ beerCount: 0 }" class="text-center">
<h1 class="text-4xl font-bold mb-8">Beer Brewing Clicker</h1>
<button @click="beerCount++" class="bg-yellow-500 hover:bg-yellow-600 text-black font-bold py-4 px-8 rounded-lg text-2xl">
Brew Beer
</button>
<div class="mt-6 text-3xl">
Beers Brewed: <span x-text="beerCount"></span>
</div>
</div>
</body>
</html>
Let’s go through the parts and take a look at what they do. I’ve pulled in Tailwind and Alpine from CDN1.
<body class="bg-gray-900 text-white flex items-center justify-center min-h-screen">
bg-gray-900 text-white
: These classes from Tailwind CSS set the background color to a dark gray and the text color to white.flex items-center justify-center
: These classes apply Flexbox layout to center the content both vertically and horizontally.min-h-screen
: This class ensures the body takes up at least the full height of the screen.
<div x-data="{ beerCount: 0 }" class="text-center">
class="text-center"
: This Tailwind CSS class centers the text within the<div>
.x-data="{ beerCount: 0 }"
: This Alpine.js directive initializes a reactive data object withbeerCount
set to 0. The{ beerCount: 0 }
is the data model that Alpine.js will manage.
<button @click="beerCount++"
@click="beerCount++"
: This Alpine.js directive binds the button click event to the function that increments thebeerCount
variable by 1.class="bg-yellow-500 hover:bg-yellow-600 text-black font-bold py-4 px-8 rounded-lg text-2xl"
: This Tailwind CSS class styles the button with a yellow background, a hover effect that changes the background to a slightly darker yellow, black text, padding, rounded corners, and large text.
<span x-text="beerCount"></span>
x-text="beerCount"
: This Alpine.js directive binds the content of the<span>
to thebeerCount
variable, dynamically updating the displayed number of beers brewed.
In this example, Alpine.js is used to add simple interactivity to the Beer Brewing Clicker game. Alpine.js manages the beerCount
variable, which starts at zero and increments each time the “Brew Beer” button is clicked. The x-data
directive initializes the beerCount
as a reactive data property, while the @click
directive binds the button’s click event to a function that increases the count. The x-text
directive automatically updates the displayed number of beers brewed, reflecting the current value of beerCount
in real-time.
You can now try the code. Every time you click on “Brew Beer” you’ll see that the “Beers Brewed” part will increase by one.
To try out this code go here, or download the code here.
Next time, we’ll add an upgrade to increase the number of beers per click.
- Content distribution network; content delivery network. ↩︎